This is
MongoDB
MongoDB is a powerful and flexible NoSQL database that is designed to handle large volumes of data and provide high performance and scalability. It is known for its document-oriented data model, dynamic schema, and rich querying capabilities. Let's explore MongoDB and its features in detail.
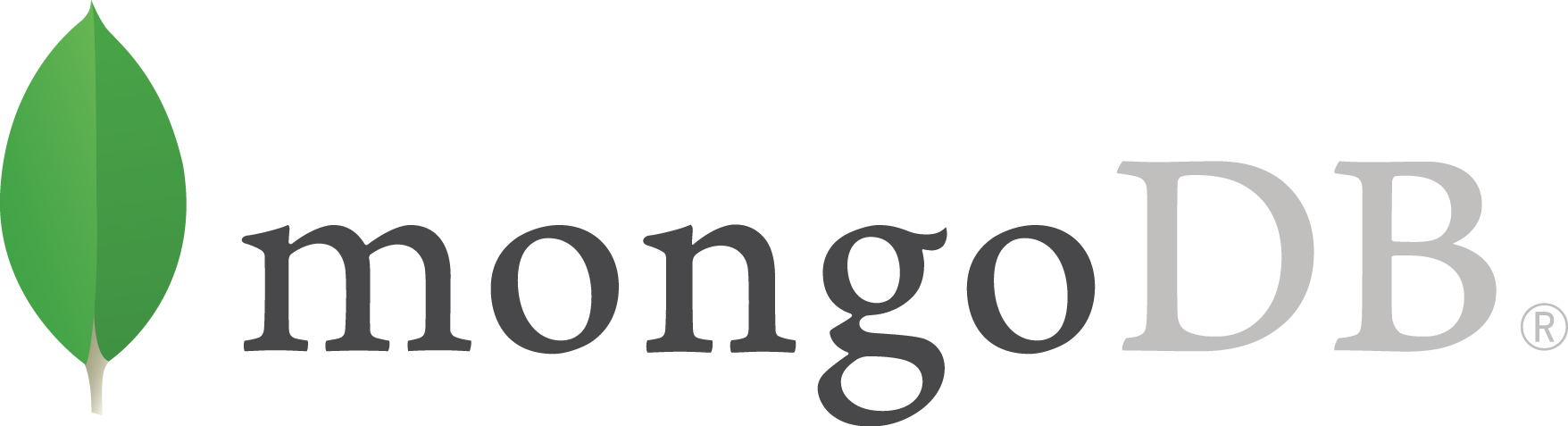
Document-Oriented Data Model
MongoDB stores data in flexible, JSON-like documents, making it well-suited for handling diverse and evolving data structures. Each document can have its own unique schema, allowing developers to store and query data in a way that best fits their application's needs.
Example: MongoDB Document
Here's an example of a document stored in MongoDB:
{
"_id": ObjectId("5e9b3b2ffbc83e38713d02f7"),
"name": "John Doe",
"age": 30,
"email": "john@example.com",
"address": {
"street": "123 Main St",
"city": "New York",
"state": "NY"
}
}
In this example, we have a document representing a person with fields for name, age, email, and address. The address field is nested within the document, demonstrating the flexibility of MongoDB's document-oriented data model.
Rich Querying and Indexing
MongoDB provides powerful querying capabilities, including support for ad-hoc queries, aggregation pipelines, and geospatial queries. Additionally, MongoDB supports indexing, which allows developers to optimize query performance and improve the efficiency of data retrieval operations.
Scalability and High Availability
MongoDB is designed to scale horizontally, allowing it to handle large volumes of data and high throughput workloads with ease. It supports sharding, replication, and distributed architectures, enabling developers to build highly available and resilient applications.
Example: Running MongoDB Queries
Let's execute a simple query in MongoDB to find all documents with the age field greater than 25:
// Connect to MongoDB server
const MongoClient = require('mongodb').MongoClient;
const uri = 'mongodb://localhost:27017';
const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true });
// Find documents with age > 25
client.connect((err, client) => {
if (err) throw err;
const db = client.db('mydatabase');
const collection = db.collection('mycollection');
collection.find({ age: { $gt: 25 } }).toArray((err, docs) => {
if (err) throw err;
console.log('Documents with age > 25:', docs);
client.close();
});
});
This code connects to a MongoDB server, retrieves a collection, and finds all documents with the age field greater than 25 using a query.
Community and Support
MongoDB has a thriving community of developers, contributors, and users who actively contribute to its development and ecosystem. The MongoDB community provides extensive documentation, tutorials, and resources to help developers learn and leverage the power of MongoDB effectively.
Conclusion
MongoDB is a versatile and feature-rich NoSQL database that provides developers with the tools they need to build scalable, high-performance applications. With its document-oriented data model, powerful querying capabilities, and support for scalability and high availability, MongoDB is a popular choice for modern web and mobile applications.