This is
Node.js
Node.js is a powerful runtime environment that allows developers to build scalable and high-performance server-side applications using JavaScript. It is built on the V8 JavaScript engine, the same engine that powers Google Chrome, and provides a non-blocking, event-driven architecture that makes it well-suited for building real-time web applications. Let's explore the features and capabilities of Node.js in detail.
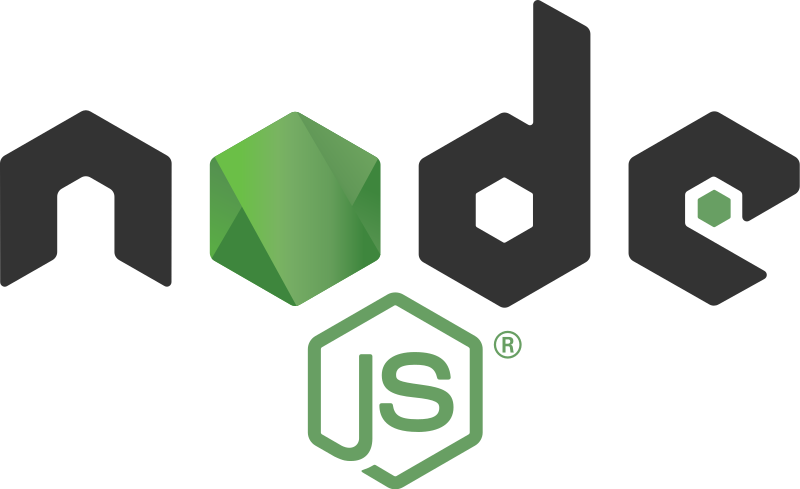
Event-Driven Architecture
Node.js employs an event-driven, non-blocking I/O model, which allows it to handle multiple concurrent connections efficiently. This architecture enables Node.js to process incoming requests asynchronously, making it ideal for building real-time applications such as chat applications, online gaming platforms, and streaming services.
Asynchronous Programming with Callbacks, Promises, and Async/Await
Node.js utilizes asynchronous programming techniques to handle I/O operations without blocking the execution of other code. Developers can use callbacks, promises, and async/await syntax to manage asynchronous operations and handle errors effectively.
Example: Asynchronous File I/O
Let's illustrate asynchronous file I/O in Node.js using the fs module:
const fs = require('fs');
// Asynchronous file read operation
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File content:', data);
});
In this example, the readFile function asynchronously reads the contents of a file and invokes a callback function once the operation is complete.
Modular and Extensible
Node.js follows a modular approach, allowing developers to split their applications into smaller, reusable modules. This modular architecture fosters code reusability, maintainability, and scalability. Additionally, Node.js has a rich ecosystem of npm (Node Package Manager) modules, providing access to thousands of pre-built packages for various functionalities.
Scalability and Performance
Node.js is designed for high concurrency and low latency, making it well-suited for building scalable and high-performance applications. Its event-driven architecture and non-blocking I/O model enable it to handle large volumes of concurrent connections efficiently, making it an ideal choice for building web servers, APIs, and microservices.
Example: Creating a Simple HTTP Server
Let's create a simple HTTP server using Node.js:
const http = require('http');
// Create a HTTP server
const server = http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, World!\n');
});
// Listen on port 3000
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
This code creates an HTTP server that listens on port 3000 and responds with "Hello, World!" to incoming HTTP requests.
Community and Support
Node.js has a vibrant and active community of developers, contributors, and enthusiasts who actively contribute to its development and ecosystem. The Node.js community provides extensive documentation, tutorials, and resources to help developers learn and leverage the power of Node.js effectively.
Conclusion
Node.js revolutionizes server-side development with its event-driven architecture, asynchronous programming model, and rich ecosystem of modules. Whether building real-time web applications, APIs, or microservices, Node.js empowers developers to build scalable, high-performance applications with ease.